Commit 589e1ad37b799f0202a73be86b3d0ce70f84231c
Committed by
jcartign
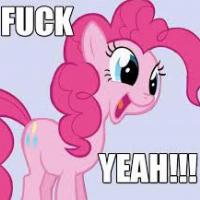
0 parents
First version
Showing
17 changed files
with
458 additions
and
0 deletions
Show diff stats
1 | +++ a/pom.xml | |
... | ... | @@ -0,0 +1,37 @@ |
1 | +<?xml version="1.0" encoding="UTF-8"?> | |
2 | +<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> | |
3 | + <modelVersion>4.0.0</modelVersion> | |
4 | + <groupId>fr.plil.gis.sio</groupId> | |
5 | + <artifactId>examen</artifactId> | |
6 | + <version>0.1</version> | |
7 | + | |
8 | + <parent> | |
9 | + <groupId>org.springframework.boot</groupId> | |
10 | + <artifactId>spring-boot-starter-parent</artifactId> | |
11 | + <version>1.4.1.RELEASE</version> | |
12 | + <relativePath/> | |
13 | + </parent> | |
14 | + | |
15 | + <properties> | |
16 | + <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> | |
17 | + <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> | |
18 | + <java.version>1.8</java.version> | |
19 | + </properties> | |
20 | + | |
21 | + <dependencies> | |
22 | + <dependency> | |
23 | + <groupId>org.springframework.boot</groupId> | |
24 | + <artifactId>spring-boot-starter-data-jpa</artifactId> | |
25 | + </dependency> | |
26 | + <dependency> | |
27 | + <groupId>com.h2database</groupId> | |
28 | + <artifactId>h2</artifactId> | |
29 | + </dependency> | |
30 | + <dependency> | |
31 | + <groupId>org.springframework.boot</groupId> | |
32 | + <artifactId>spring-boot-starter-test</artifactId> | |
33 | + <scope>test</scope> | |
34 | + </dependency> | |
35 | + </dependencies> | |
36 | + | |
37 | +</project> | ... | ... |
src/main/java/fr/plil/sio/examen/ExamenApplication.java
0 → 100644
1 | +++ a/src/main/java/fr/plil/sio/examen/api/Animal.java | |
... | ... | @@ -0,0 +1,52 @@ |
1 | +package fr.plil.sio.examen.api; | |
2 | + | |
3 | +import java.io.Serializable; | |
4 | +import javax.persistence.Column; | |
5 | +import javax.persistence.Entity; | |
6 | +import javax.persistence.GeneratedValue; | |
7 | +import javax.persistence.GenerationType; | |
8 | +import javax.persistence.Id; | |
9 | +import javax.persistence.ManyToOne; | |
10 | + | |
11 | +/** | |
12 | + * An animal must have a name one and only one owner | |
13 | + */ | |
14 | +@Entity | |
15 | +public class Animal implements Serializable { | |
16 | + | |
17 | + @Id | |
18 | + @GeneratedValue(strategy = GenerationType.AUTO) | |
19 | + private Long id; | |
20 | + | |
21 | + @Column(nullable = false) | |
22 | + private String name; | |
23 | + | |
24 | + @ManyToOne(optional = false) | |
25 | + private Owner owner; | |
26 | + | |
27 | + public Long getId() { | |
28 | + return id; | |
29 | + } | |
30 | + | |
31 | + public void setId(Long id) { | |
32 | + this.id = id; | |
33 | + } | |
34 | + | |
35 | + public String getName() { | |
36 | + return name; | |
37 | + } | |
38 | + | |
39 | + public void setName(String name) { | |
40 | + this.name = name; | |
41 | + } | |
42 | + | |
43 | + public Owner getOwner() { | |
44 | + return owner; | |
45 | + } | |
46 | + | |
47 | + public void setOwner(Owner owner) { | |
48 | + this.owner = owner; | |
49 | + } | |
50 | + | |
51 | + | |
52 | +} | ... | ... |
1 | +++ a/src/main/java/fr/plil/sio/examen/api/Comment.java | |
... | ... | @@ -0,0 +1,21 @@ |
1 | +package fr.plil.sio.examen.api; | |
2 | + | |
3 | +/** | |
4 | + * A comment is made by an reporter (i.e. an owner) on ANY animal | |
5 | + * (not only the ones he owns). | |
6 | + * An reporter can have zero, one or more comments on any animal (i.e. it can | |
7 | + * exists several comments from the same reporter on the same animal). | |
8 | + * An animal can have zero, one or more comments by any reporter (i.e. it can | |
9 | + * exists several comments from the same reporter on the same animal). | |
10 | + * | |
11 | + * TODO: complete the classes in API to support comment serialization in the | |
12 | + * database. | |
13 | + */ | |
14 | +public class Comment { | |
15 | + | |
16 | + private Owner reporter; | |
17 | + | |
18 | + private Animal animal; | |
19 | + | |
20 | + private String message; | |
21 | +} | ... | ... |
1 | +++ a/src/main/java/fr/plil/sio/examen/api/Owner.java | |
... | ... | @@ -0,0 +1,51 @@ |
1 | +package fr.plil.sio.examen.api; | |
2 | + | |
3 | +import java.util.LinkedList; | |
4 | +import java.util.List; | |
5 | +import javax.persistence.Column; | |
6 | +import javax.persistence.Entity; | |
7 | +import javax.persistence.GeneratedValue; | |
8 | +import javax.persistence.GenerationType; | |
9 | +import javax.persistence.Id; | |
10 | +import javax.persistence.OneToMany; | |
11 | + | |
12 | +/** | |
13 | + * An owner has a name and zero, one or more animals. | |
14 | + */ | |
15 | +@Entity | |
16 | +public class Owner { | |
17 | + | |
18 | + @Id | |
19 | + @GeneratedValue(strategy = GenerationType.AUTO) | |
20 | + private Long id; | |
21 | + | |
22 | + @Column(nullable = false) | |
23 | + private String name; | |
24 | + | |
25 | + @OneToMany(mappedBy = "owner") | |
26 | + private List<Animal> animals = new LinkedList<>(); | |
27 | + | |
28 | + public Long getId() { | |
29 | + return id; | |
30 | + } | |
31 | + | |
32 | + public void setId(Long id) { | |
33 | + this.id = id; | |
34 | + } | |
35 | + | |
36 | + public String getName() { | |
37 | + return name; | |
38 | + } | |
39 | + | |
40 | + public void setName(String name) { | |
41 | + this.name = name; | |
42 | + } | |
43 | + | |
44 | + public List<Animal> getAnimals() { | |
45 | + return animals; | |
46 | + } | |
47 | + | |
48 | + public void setAnimals(List<Animal> animals) { | |
49 | + this.animals = animals; | |
50 | + } | |
51 | +} | ... | ... |
src/main/java/fr/plil/sio/examen/repositories/AnimalRepository.java
0 → 100644
1 | +++ a/src/main/java/fr/plil/sio/examen/repositories/AnimalRepository.java | |
... | ... | @@ -0,0 +1,15 @@ |
1 | +package fr.plil.sio.examen.repositories; | |
2 | + | |
3 | +import fr.plil.sio.examen.api.Animal; | |
4 | +import fr.plil.sio.examen.api.Owner; | |
5 | +import java.util.List; | |
6 | +import org.springframework.data.jpa.repository.JpaRepository; | |
7 | + | |
8 | +public interface AnimalRepository extends JpaRepository<Animal,Long> { | |
9 | + | |
10 | + /** | |
11 | + * NOTE: this is an important example to help you with the findByXXX methods | |
12 | + * in the comment repository. | |
13 | + */ | |
14 | + List<Animal> findByOwner(Owner owner); | |
15 | +} | ... | ... |
src/main/java/fr/plil/sio/examen/repositories/CommentRepository.java
0 → 100644
1 | +++ a/src/main/java/fr/plil/sio/examen/repositories/CommentRepository.java | |
... | ... | @@ -0,0 +1,10 @@ |
1 | +package fr.plil.sio.examen.repositories; | |
2 | + | |
3 | +import fr.plil.sio.examen.api.Comment; | |
4 | +import org.springframework.data.jpa.repository.JpaRepository; | |
5 | + | |
6 | +/** | |
7 | + * TODO: complete this interface for new find methods. | |
8 | + */ | |
9 | +public interface CommentRepository { //extends JpaRepository<Comment, Long>{ | |
10 | +} | ... | ... |
src/main/java/fr/plil/sio/examen/repositories/OwnerRepository.java
0 → 100644
1 | +++ a/src/main/java/fr/plil/sio/examen/repositories/OwnerRepository.java | |
... | ... | @@ -0,0 +1,8 @@ |
1 | +package fr.plil.sio.examen.repositories; | |
2 | + | |
3 | +import fr.plil.sio.examen.api.Owner; | |
4 | +import org.springframework.data.jpa.repository.JpaRepository; | |
5 | + | |
6 | +public interface OwnerRepository extends JpaRepository<Owner, Long>{ | |
7 | + | |
8 | +} | ... | ... |
src/main/java/fr/plil/sio/examen/services/AnimalService.java
0 → 100644
1 | +++ a/src/main/java/fr/plil/sio/examen/services/AnimalService.java | |
... | ... | @@ -0,0 +1,19 @@ |
1 | +package fr.plil.sio.examen.services; | |
2 | + | |
3 | +import fr.plil.sio.examen.api.Animal; | |
4 | +import fr.plil.sio.examen.api.Owner; | |
5 | + | |
6 | +public interface AnimalService { | |
7 | + | |
8 | + /** | |
9 | + * Create an animal attached to an owner | |
10 | + */ | |
11 | + Animal create(String name, Owner owner); | |
12 | + | |
13 | + /** | |
14 | + * Delete an animal and ALL comment associated. | |
15 | + * | |
16 | + * TODO: complete this method. | |
17 | + */ | |
18 | + void delete(Animal animal); | |
19 | +} | ... | ... |
src/main/java/fr/plil/sio/examen/services/AnimalServiceImpl.java
0 → 100644
1 | +++ a/src/main/java/fr/plil/sio/examen/services/AnimalServiceImpl.java | |
... | ... | @@ -0,0 +1,39 @@ |
1 | +package fr.plil.sio.examen.services; | |
2 | + | |
3 | +import fr.plil.sio.examen.api.Animal; | |
4 | +import fr.plil.sio.examen.api.Owner; | |
5 | +import fr.plil.sio.examen.repositories.AnimalRepository; | |
6 | +import org.springframework.beans.factory.annotation.Autowired; | |
7 | +import org.springframework.stereotype.Service; | |
8 | +import org.springframework.transaction.annotation.Transactional; | |
9 | + | |
10 | +@Service | |
11 | +public class AnimalServiceImpl implements AnimalService { | |
12 | + | |
13 | + @Autowired | |
14 | + private AnimalRepository animalRepository; | |
15 | + | |
16 | + @Override | |
17 | + @Transactional | |
18 | + public Animal create(String name, Owner owner) { | |
19 | + if(name == null) { | |
20 | + throw new IllegalArgumentException("name must be not null"); | |
21 | + } | |
22 | + if(owner == null) { | |
23 | + throw new IllegalArgumentException("owner must be not null"); | |
24 | + } | |
25 | + Animal animal = new Animal(); | |
26 | + animal.setName(name); | |
27 | + animal.setOwner(owner); | |
28 | + animalRepository.save(animal); | |
29 | + return animal; | |
30 | + } | |
31 | + | |
32 | + | |
33 | + @Override | |
34 | + @Transactional | |
35 | + public void delete(Animal animal) { | |
36 | + throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. | |
37 | + } | |
38 | + | |
39 | +} | ... | ... |
src/main/java/fr/plil/sio/examen/services/CommentService.java
0 → 100644
1 | +++ a/src/main/java/fr/plil/sio/examen/services/CommentService.java | |
... | ... | @@ -0,0 +1,34 @@ |
1 | +package fr.plil.sio.examen.services; | |
2 | + | |
3 | +import fr.plil.sio.examen.api.Animal; | |
4 | +import fr.plil.sio.examen.api.Comment; | |
5 | +import fr.plil.sio.examen.api.Owner; | |
6 | +import java.util.List; | |
7 | + | |
8 | +public interface CommentService { | |
9 | + | |
10 | + /** | |
11 | + * Add a new comment (message) made by a reporter to an animal | |
12 | + */ | |
13 | + Comment add(Owner reporter, Animal animal, String message); | |
14 | + | |
15 | + /** | |
16 | + * Delete the comment | |
17 | + */ | |
18 | + void delete(Comment comment); | |
19 | + | |
20 | + /** | |
21 | + * List all messages about an animal | |
22 | + */ | |
23 | + List<Comment> findByAnimal(Animal animal); | |
24 | + | |
25 | + /** | |
26 | + * List all messages reported by one owner | |
27 | + */ | |
28 | + List<Comment> findByReporter(Owner reporter); | |
29 | + | |
30 | + /** | |
31 | + * List all messages about all animals of one owner | |
32 | + */ | |
33 | + List<Comment> findByOwner(Owner owner); | |
34 | +} | ... | ... |
src/main/java/fr/plil/sio/examen/services/CommentServiceImpl.java
0 → 100644
1 | +++ a/src/main/java/fr/plil/sio/examen/services/CommentServiceImpl.java | |
... | ... | @@ -0,0 +1,46 @@ |
1 | +package fr.plil.sio.examen.services; | |
2 | + | |
3 | +import fr.plil.sio.examen.api.Animal; | |
4 | +import fr.plil.sio.examen.api.Comment; | |
5 | +import fr.plil.sio.examen.api.Owner; | |
6 | +import java.util.List; | |
7 | +import org.springframework.stereotype.Service; | |
8 | +import org.springframework.transaction.annotation.Transactional; | |
9 | + | |
10 | +/** | |
11 | + * TODO: complete all these methods | |
12 | + */ | |
13 | +@Service | |
14 | +public class CommentServiceImpl implements CommentService { | |
15 | + | |
16 | + @Override | |
17 | + @Transactional | |
18 | + public Comment add(Owner owner, Animal animal, String text) { | |
19 | + throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. | |
20 | + } | |
21 | + | |
22 | + @Override | |
23 | + @Transactional | |
24 | + public void delete(Comment comment) { | |
25 | + throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. | |
26 | + } | |
27 | + | |
28 | + @Override | |
29 | + @Transactional(readOnly = true) | |
30 | + public List<Comment> findByAnimal(Animal animal) { | |
31 | + throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. | |
32 | + } | |
33 | + | |
34 | + @Override | |
35 | + @Transactional(readOnly = true) | |
36 | + public List<Comment> findByReporter(Owner reporter) { | |
37 | + throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. | |
38 | + } | |
39 | + | |
40 | + @Override | |
41 | + @Transactional(readOnly = true) | |
42 | + public List<Comment> findByOwner(Owner owner) { | |
43 | + throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. | |
44 | + } | |
45 | + | |
46 | +} | ... | ... |
src/main/java/fr/plil/sio/examen/services/OwnerService.java
0 → 100644
1 | +++ a/src/main/java/fr/plil/sio/examen/services/OwnerService.java | |
... | ... | @@ -0,0 +1,18 @@ |
1 | +package fr.plil.sio.examen.services; | |
2 | + | |
3 | +import fr.plil.sio.examen.api.Owner; | |
4 | + | |
5 | +public interface OwnerService { | |
6 | + | |
7 | + /** | |
8 | + * Create an owner | |
9 | + */ | |
10 | + Owner create(String name); | |
11 | + | |
12 | + /** | |
13 | + * Delete an owner and ALL comment reporter by him/her. | |
14 | + * | |
15 | + * TODO: complete this method. | |
16 | + */ | |
17 | + void delete(Owner owner); | |
18 | +} | ... | ... |
src/main/java/fr/plil/sio/examen/services/OwnerServiceImpl.java
0 → 100644
1 | +++ a/src/main/java/fr/plil/sio/examen/services/OwnerServiceImpl.java | |
... | ... | @@ -0,0 +1,30 @@ |
1 | +package fr.plil.sio.examen.services; | |
2 | + | |
3 | +import fr.plil.sio.examen.api.Owner; | |
4 | +import fr.plil.sio.examen.repositories.OwnerRepository; | |
5 | +import org.springframework.beans.factory.annotation.Autowired; | |
6 | +import org.springframework.stereotype.Service; | |
7 | + | |
8 | +@Service | |
9 | +public class OwnerServiceImpl implements OwnerService { | |
10 | + | |
11 | + @Autowired | |
12 | + private OwnerRepository ownerRepository; | |
13 | + | |
14 | + @Override | |
15 | + public void delete(Owner owner) { | |
16 | + throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. | |
17 | + } | |
18 | + | |
19 | + @Override | |
20 | + public Owner create(String name) { | |
21 | + if(name == null) { | |
22 | + throw new IllegalArgumentException("name must be not null"); | |
23 | + } | |
24 | + Owner owner = new Owner(); | |
25 | + owner.setName(name); | |
26 | + ownerRepository.save(owner); | |
27 | + return owner; | |
28 | + } | |
29 | + | |
30 | +} | ... | ... |
src/test/java/fr/plil/sio/examen/AnimalRepositoryTest.java
0 → 100644
1 | +++ a/src/test/java/fr/plil/sio/examen/AnimalRepositoryTest.java | |
... | ... | @@ -0,0 +1,36 @@ |
1 | +package fr.plil.sio.examen; | |
2 | + | |
3 | +import fr.plil.sio.examen.api.Animal; | |
4 | +import fr.plil.sio.examen.api.Owner; | |
5 | +import fr.plil.sio.examen.repositories.AnimalRepository; | |
6 | +import fr.plil.sio.examen.repositories.OwnerRepository; | |
7 | +import org.junit.Test; | |
8 | +import org.junit.runner.RunWith; | |
9 | +import org.springframework.beans.factory.annotation.Autowired; | |
10 | +import org.springframework.boot.test.context.SpringBootTest; | |
11 | +import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; | |
12 | +import org.springframework.transaction.annotation.Transactional; | |
13 | + | |
14 | +@RunWith(SpringJUnit4ClassRunner.class) | |
15 | +@SpringBootTest | |
16 | +@Transactional | |
17 | +public class AnimalRepositoryTest { | |
18 | + | |
19 | + @Autowired | |
20 | + private AnimalRepository animalRepository; | |
21 | + | |
22 | + @Autowired | |
23 | + private OwnerRepository ownerRepository; | |
24 | + | |
25 | + @Test | |
26 | + public void testSimpleOperations() { | |
27 | + Owner owner = new Owner(); | |
28 | + owner.setName("mickey"); | |
29 | + ownerRepository.save(owner); | |
30 | + Animal animal = new Animal(); | |
31 | + animal.setName("pluto"); | |
32 | + animal.setOwner(owner); | |
33 | + owner.getAnimals().add(animal); | |
34 | + animalRepository.save(animal); | |
35 | + } | |
36 | +} | ... | ... |
src/test/java/fr/plil/sio/examen/AnimalServiceTest.java
0 → 100644
1 | +++ a/src/test/java/fr/plil/sio/examen/AnimalServiceTest.java | |
... | ... | @@ -0,0 +1,29 @@ |
1 | +package fr.plil.sio.examen; | |
2 | + | |
3 | +import fr.plil.sio.examen.api.Owner; | |
4 | +import fr.plil.sio.examen.services.AnimalService; | |
5 | +import fr.plil.sio.examen.services.OwnerService; | |
6 | +import org.junit.Test; | |
7 | +import org.junit.runner.RunWith; | |
8 | +import org.springframework.beans.factory.annotation.Autowired; | |
9 | +import org.springframework.boot.test.context.SpringBootTest; | |
10 | +import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; | |
11 | +import org.springframework.transaction.annotation.Transactional; | |
12 | + | |
13 | +@RunWith(SpringJUnit4ClassRunner.class) | |
14 | +@SpringBootTest | |
15 | +@Transactional | |
16 | +public class AnimalServiceTest { | |
17 | + | |
18 | + @Autowired | |
19 | + private AnimalService animalService; | |
20 | + | |
21 | + @Autowired | |
22 | + private OwnerService ownerService; | |
23 | + | |
24 | + @Test | |
25 | + public void createAnimalAndOwner() { | |
26 | + Owner o = ownerService.create("owner"); | |
27 | + animalService.create("animal", o); | |
28 | + } | |
29 | +} | ... | ... |
1 | +++ a/src/test/resources/application.properties | |
... | ... | @@ -0,0 +1,5 @@ |
1 | +logging.level.org.springframework=INFO | |
2 | +logging.level.org.hibernate.SQL=DEBUG | |
3 | +logging.level.org.hibernate=INFO | |
4 | +spring.datasource.url=jdbc:h2:mem:persistence;TRACE_LEVEL_FILE=4;DB_CLOSE_ON_EXIT=FALSE | |
5 | +spring.datasource.tomcat.max-active=500 | ... | ... |